TensorRT部署YOLOv5 06 视频推理
在上一篇文章中,对使用TensorRT引擎进行单幅图像推理进行了介绍,本文仍然使用Python API,在上篇文章的基础上,对视频实时推理的实现进行介绍
workflow
视频实时推理的过程大致如下
加载TensorRT引擎文件并进行反序列化,创建执行上下文context
根据引擎申请输入输出Buffers,并进行绑定
读入一帧图像数据
图像预处理
调用推断API进行推断
输出解码
画框并显示
返回步骤3
这里从视频文件读入图像数据以及画框显示使用的都是opencv
实现
大部分代码处理与上一篇文章相同,视频推理的整个程序主体如下
1 | with get_engine(args.engine, TRT_LOGGER) as engine: \ |
运行该程序,加载自己训练的yolov5m的20分类模型,结果帧率只有1fps+,在TensorRT引擎转换阶段,是可以大致清楚一个模型的推理延迟的,而目前的帧率只有1fps显然过于低了。该程序中使用opencv进行h.264视频的加载和解码,使用的是软解码,实时性能会比较差,Nvidia开发平台内置了硬件h264编解码器,而该编解码加速器适配到了Gstreamer,因此在上层应用中,使用opencv时可以构建Gstreamer的pipeline,将视频传送给硬件编解码器进行解码,然后再设置输出端为appsink,即当前程序接收解码后的数据
以下是构建h264的Gstreamer pipeline
1 | def video_pipeline(filesrc, width, height): |
将opencv创建Capture的代码改写一下
1 | cap = cv2.VideoCapture(video_pipeline(args.video, args.width, |
改写后运行代码,帧率提升到了3fps+,后文将继续介绍如何进行帧率提升的优化方法
运行效果
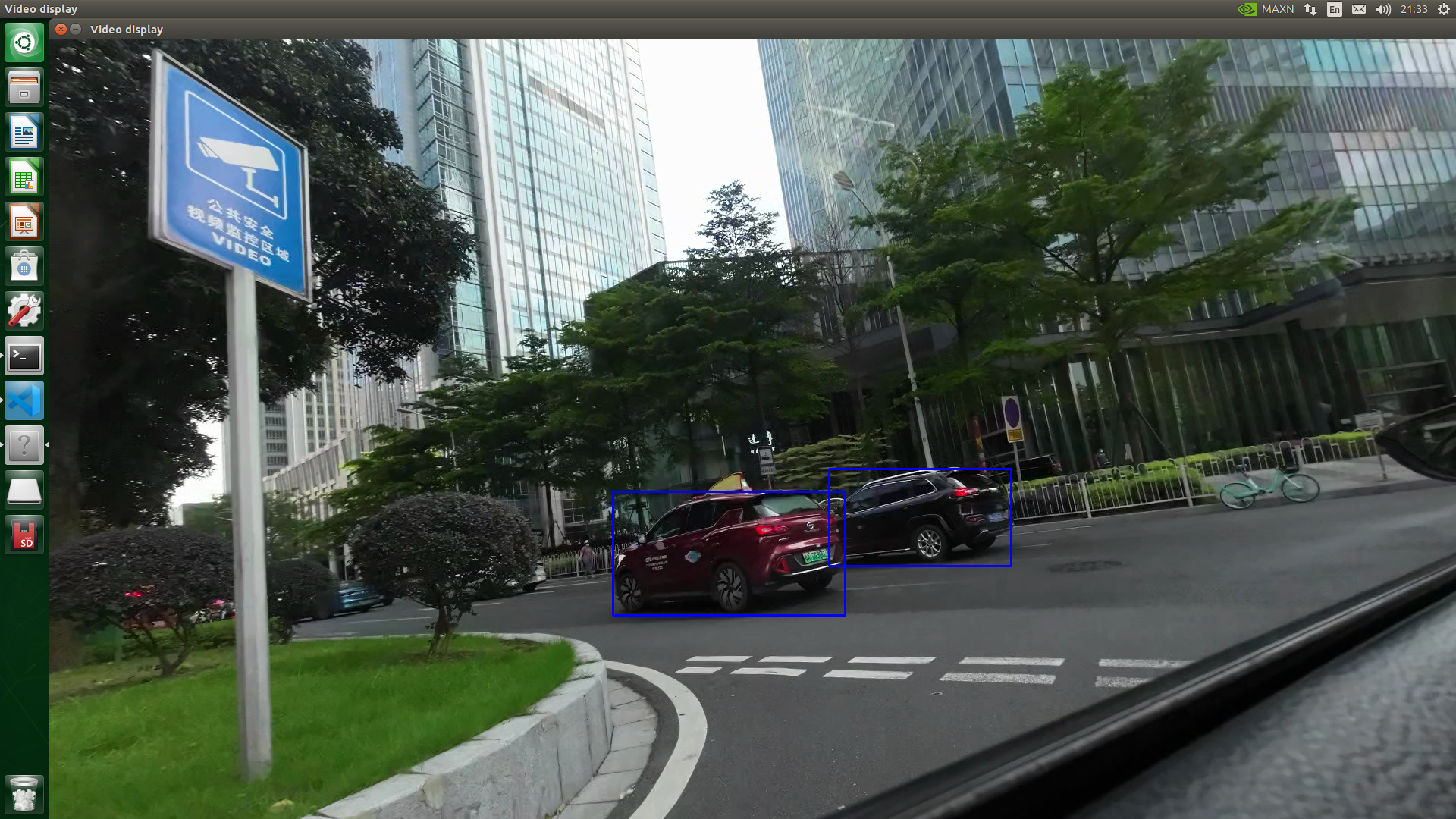